Learn How To Be a Better Programmer by Automating the Boring Stuff
A good problem provides a stage. A stage where you can apply your knowledge, see its effects, and draw conclusions from it to improve and go back to perform.
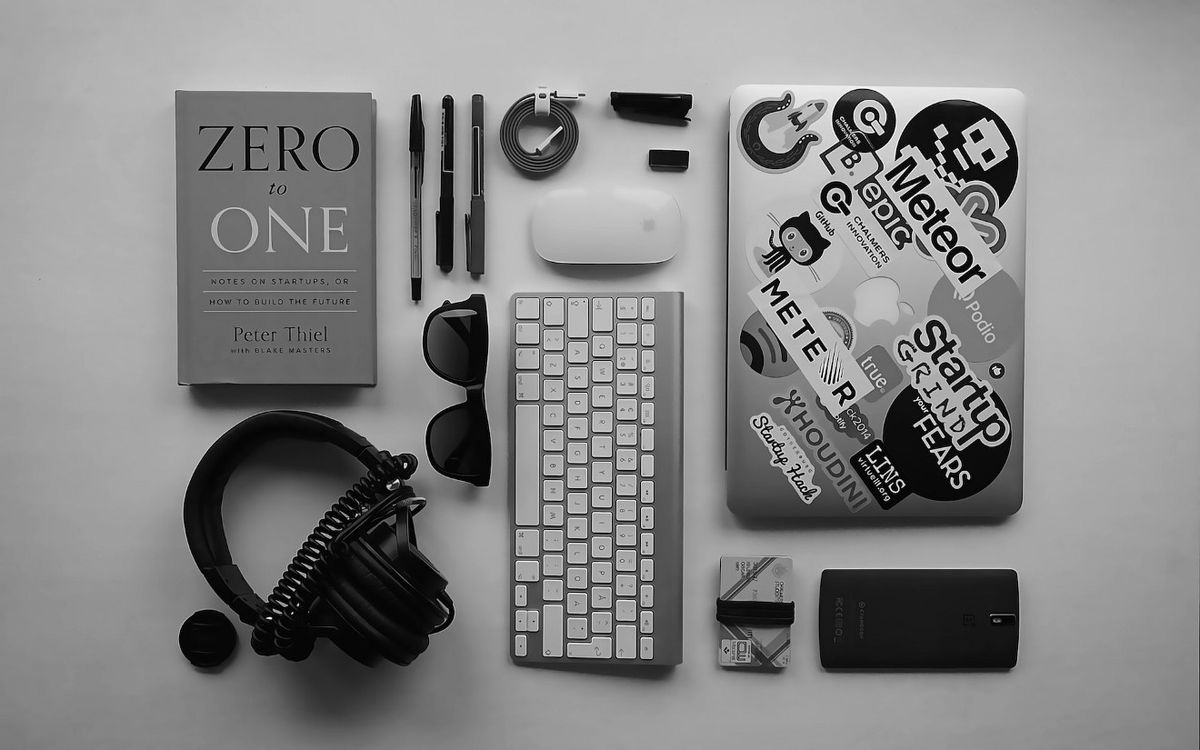
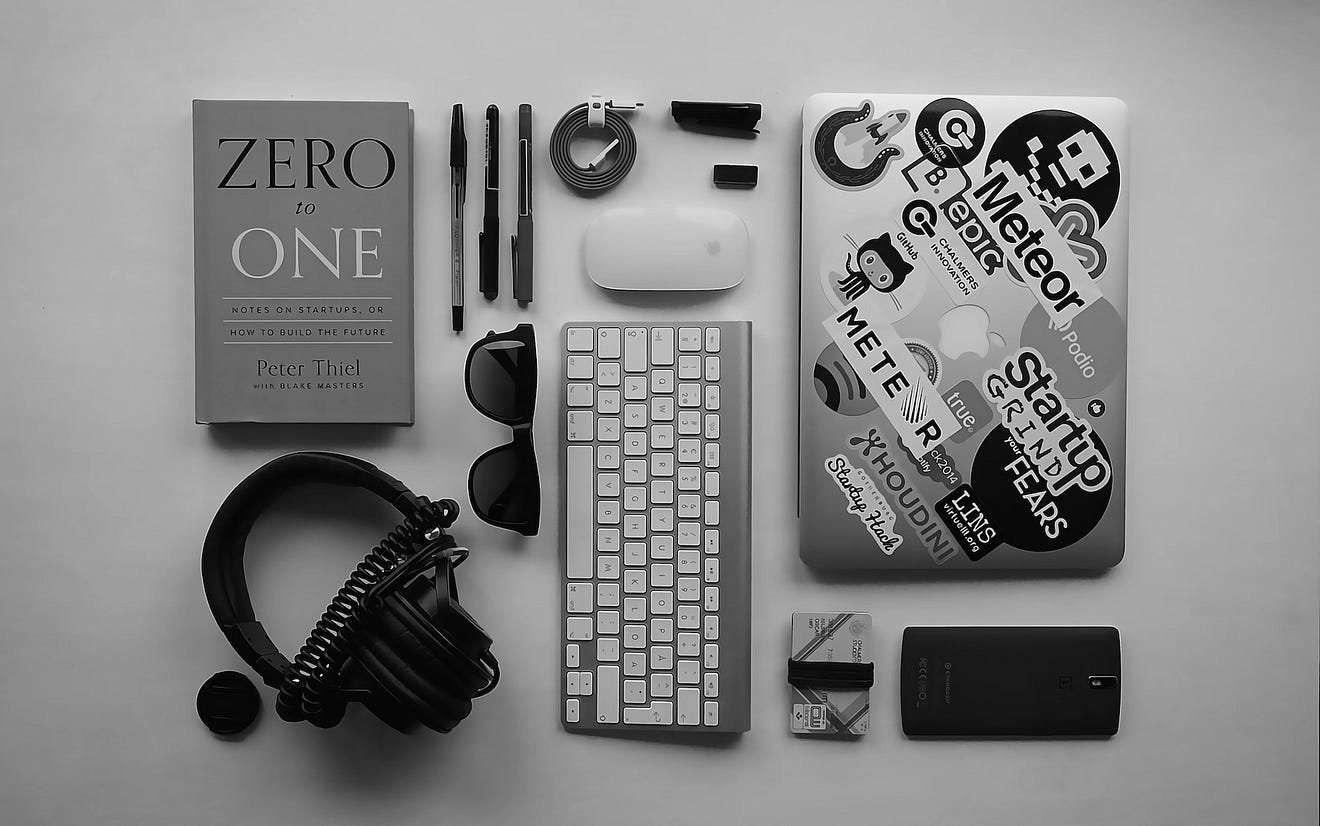
The equivalent of playing scales is writing small programs, alone. Unfortunately, playing scales (a) doesn’t teach you anything about music, and (b) is boring as hell.
This is what Eric S. Raymond said in his article, How to Learn Hacking. I have been learning programming for more than one year now, and I have to say that I agree with him.
Unfortunately, teaching-through-small-programs is the approach that most teachers, books, and college curricula take.
Although such programs are able to teach you the mechanics of how conditional statements and loops work and how to write functions, they fail to provide a deeper understanding of them.
The problem with the teaching-through-small-programs approach is that it doesn’t
- develop your intuition - like how to go about modularizing your code
- address how to write functions so that can be reused when extending your program to new use cases
- talk about the trade-off between writing readable code and compact code
- provide focus on the need for a good and consistent programming style
In summary, they fail to teach the core design choices that one learns to make when creating larger programs.
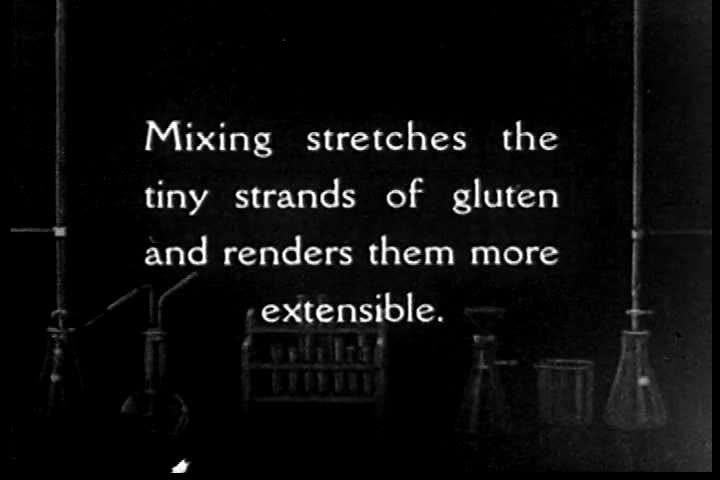
In addition to these flaws, the approach is boring as hell! Designing such features in your programs is what makes programming fun in the first place. Small programs just don’t have enough room to encourage a student to ponder over them and gain the necessary intuitions. On the other hand, with actual programs, these skills become a necessity.
This means that you must take matters into your own hands, find a good problem, and code a solution for it.
Finding good problems
A good problem provides a stage. A stage where you can apply your knowledge, see its effects, and draw conclusions from it to improve and go back to perform.
It is important that you pick a problem that you want to solve — something that seems daunting, but at the same time makes you feel like — “It would be cool if I wrote a program for this”. When the program that you are building tries to solve a problem that you care about, you will be motivated to learn and grow. You will always want to work on improving your solution.
Finding a good problem to work on might feel difficult at first. That’s only because you have set the bar too high. You may have watched the movie The Social Network too much and hope to make the next Google or Facebook out of this project. This will only slow down the learning, make you create unrealistic goals, and most dangerously, make you procrastinate.
Remember, your goal is not to write a billion dollar software. It is to create a program that is going to provide a stage for you to work on and simply learn from.
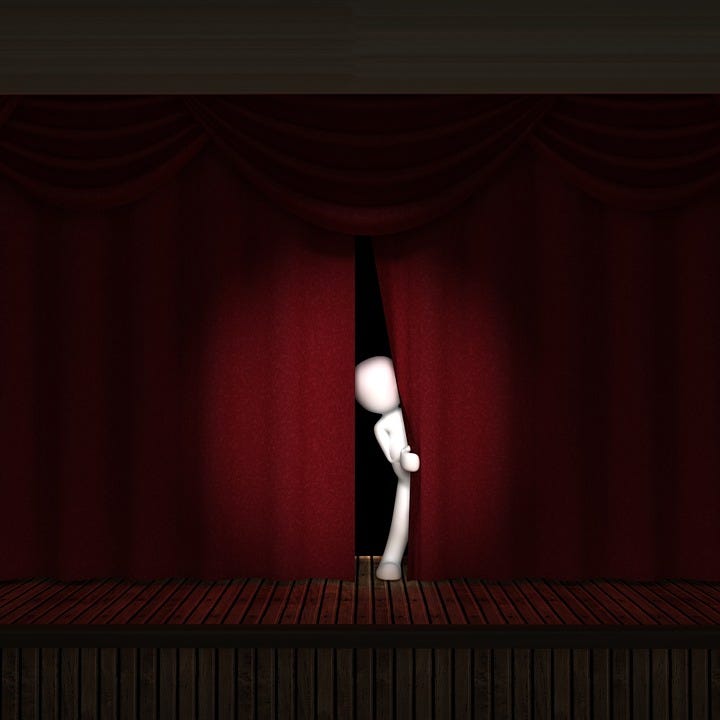
A way to find such problems
How often do you find yourself doing a task so redundant and boring that it feels like it might actually be making you dumber? How often do you wish that you had some intelligent pet or a genie who could do that work for you? And you know that they could do this work faster, too, since they wouldn’t slouch like you do.
You know what? You already have such a pet - your computer. Each of these mundane tasks presents an opportunity to write a program and tell this “intelligent pet” what it needs to do for you.
Writing good solutions
After you have chosen your problem, you will want to craft the best possible solution to it. Let’s see what Brian W. Kerninghan says about good software in his book The Practice of Programming.
The basic principles that form the bedrock of good software are simplicity, which keeps programs short and manageable; clarity, which makes sure they are easy to understand, for people as well as machines; generality, which means they work well in a broad range of situations and adapt well as new situations arise; and automation, which lets the machine do the work for us, freeing us from mundane tasks.
It is sad that only a few of the Introduction to <add programming language here> books cover these topics. It’s even sadder to think how a lot of the programming courses in colleges completely neglect this aspect of programming.
When you have a problem that you have invented (or most probably, reinvented) yourself, you will have a solution that you can care about. A solution that you want to constantly improve upon.
In this section I am going to use an example from my personal experience to better explain what I am saying.
So, I happen to use Chrome Bookmarks a lot!
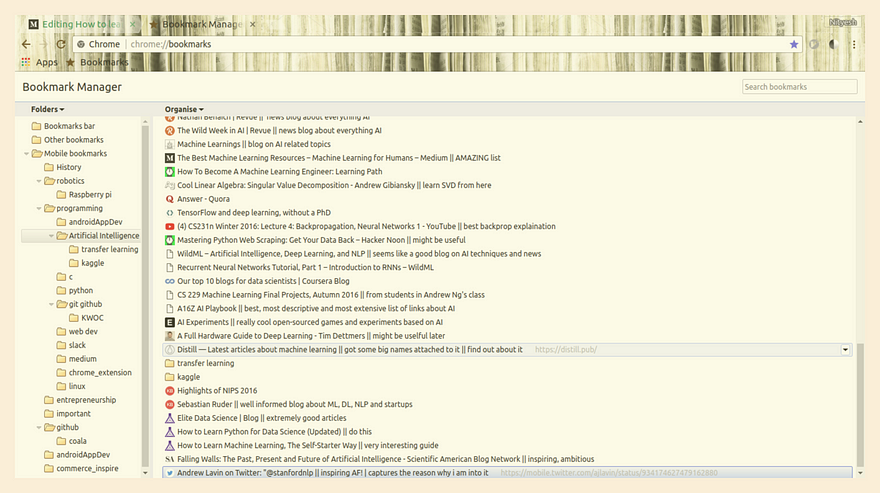
Problem 1: Adding comments to bookmarks with long titles
If you look closely, you might find that I have added personal comments at the end of these bookmarks. This is because, sometimes, it can get really difficult to remember the exact reason or motivation behind a bookmark. Sure, the title does a pretty good job at explaining what the page contains, but not why I found the contents helpful or what I feared losing had I not saved its link - which is why I added the comments.
I use ||
at the end of the default name provided by Chrome and add my comment(s) after that.
Now, here’s the problem.
Sometimes, this default name is really long and adding a comment means having to Ctrl+left
on a whole lot of words before I can get to the end. Exhausting!
I realise that the problem might not appear too severe, but it can really mess up the flow of research sometimes. Also, hey, we are not trying to create a billion dollar software, remember?
Problem 2: Copying a bookmark with its title
I bookmark every good thing I fear losing, organize it by folders depending on the topic it might belong to, and put a comment on it. For this reason, they are of great value to me. Every time someone asks me about some particular topic I know of, I try to steer the conversation so that I can share some bookmarks from that topic’s folder.
No, I am not trying to boast that I know everything or demonstrate that I am not selfish. I genuinely think that this will help them because:
- The folder contains the best of my own know-how on the subject.
- With the title and the personal comments, each bookmark becomes well documented - so the person doesn’t just get a bunch of hyperlinks and gets added value.
- I believe that this is better than me trying to spoon-feed and explain it verbally. True learning can happen only when people browse the web themselves.
Now, here’s the problem. Chrome does not provide a quick and easy way to copy a bookmark along with its title, as far as I know. If I copy
some bookmark and paste
it in some editor, I only get its hyperlink.
So, if I want to share everything, I need to go to the edit
option, select the title from there, paste it somewhere, and do the same thing for the hyperlink. And what if I want to share a whole lot of bookmarks? I need to do this for each link separately!
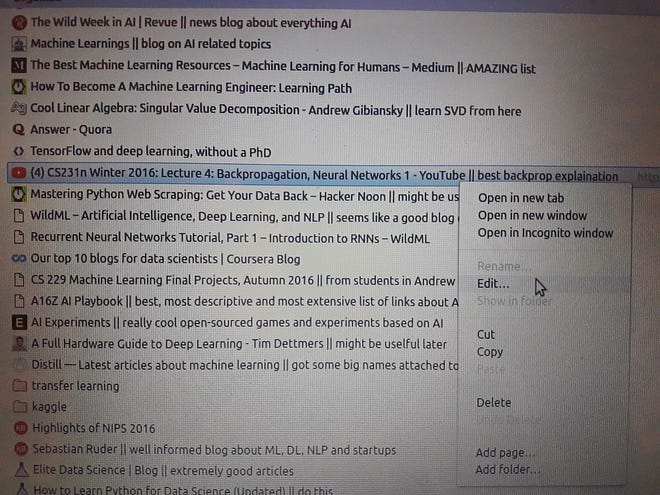
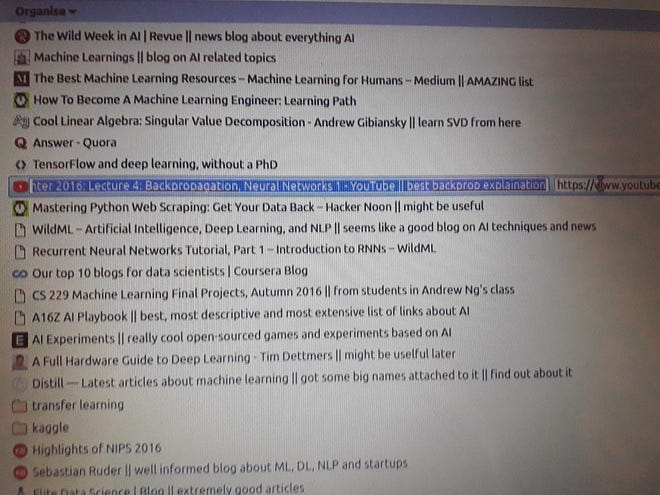
This is what I wish adding a bookmark to Chrome was like instead
- I wish that the star icon at the upper right corner of the address bar had a
Comment
option along withName
andFolder
.
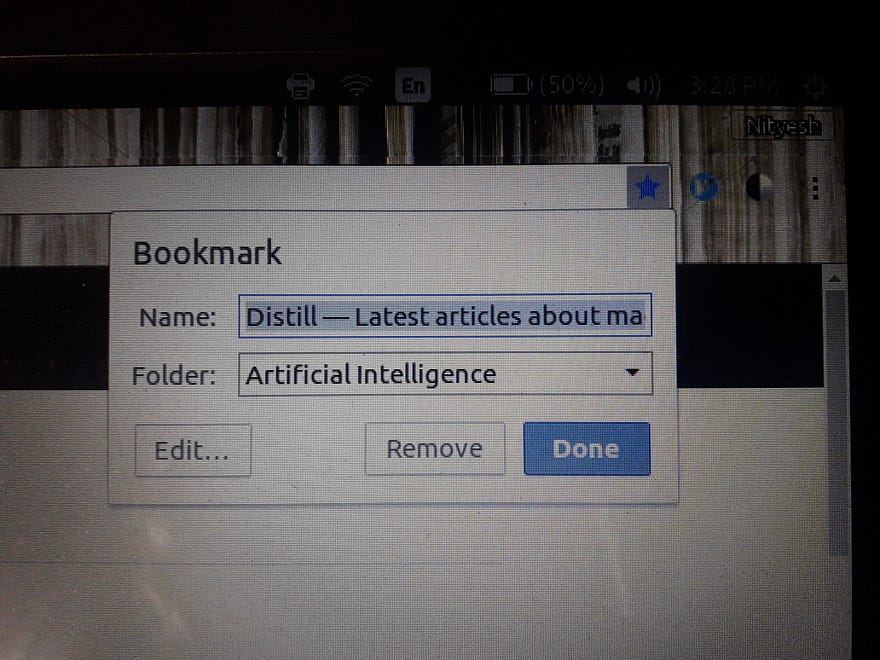
- I wish that Chrome allowed me to download all bookmarks in a folder to my local machine as a plain text file. In that file, it should display each bookmark in the following format:
* Title - <bookmark_title>
* Comment - <bookmark_comment>
* URL - <bookmark_url>
So I rubbed my magic lamp thinking, “O Genie! These are my wishes. Can you please make them come true? _/\_”
The Solution
Until last month, I did not even think that there was a plausible way to actually do that. I thought that the only way it might be possible was to use an open-source browser and tweak the code in it to suit my needs.
After some initial research I discovered that I would never be doing that. Browsers are one of the most difficult and largest pieces of software, and I am certainly not tinkering with their code no matter how welcoming the open-source community of Firefox is.
Then, I read an answer to the question “What are some great programming projects for beginners” on Quora. It said how a newbie can make a Chrome browser extension that replaces all the photos in your Facebook’s news feed to photos of cats.
That’s when I realised the power of a browser extensions and thought, that’s whats gonna help me fulfill my wishes.
Chrome browser extension and chrome.* API
The Chrome browser has a neat API called chrome.* API. That is, every API whose name starts with chrome.
Anyone can use it to create their own browser extension. Basically, it gives developers access to a few functions that they may use to easily create more abstract code.
A browser extension uses ordinary HTML/CSS along with JavaScript. I read that it’s easy, even for beginners, to create a simple browser extension. All you need is a little knowledge of HTML/CSS and JavaScript.
I had gone through the Head First HTML and CSS book a while ago, but I didn’t know any JavaScript. I thought about shying away from the project. After all, I wanted to do a project to improve my fluency with a language that I already knew (Python or C++).
But I also desperately needed a project to showcase on my Github profile.
Time to learn a new language
I decided to learn the basics of JavaScript like variable and function declarations and definitions, loops, and conditions. This hardly took any time at all. It seems like it’s basically the same thing in all the languages.
I would learn the rest of the JavaScript features as I came across them in the tutorials and guides about creating an extension. It was time to create my very own extension.
Reading the documentation and learning from it
There exists only a handful of tutorial videos or articles that can guide you to creating a Chrome browser extension. Probably because browser extensions never became too hot.
I found that the stuff that I was gonna need was present inside the chrome.bookmarks API. This turned out to be a somewhat unusual request. Relatively few extensions use it. This meant that there was no tutorial on how to use it.
Before this, everything that I ever learned was inside nicely packaged tutorials or guides that would spoon-feed the topic. But this time, I had to learn by going through the documentation only.
It was like I was presented with this whole arsenal of methods in front of me along with a small user reference manual. Now, I had to identify, by myself, the tools that could be of most help in accomplishing my goal.
In doing all this, I realized the importance of the “Hello World” program
I had always felt that the “Hello World program” was highly overrated.
I mean it is kind of welcoming to newcomers and everything, but it’s not so great that every programming book/tutorial ever needs to start with it.
It never fascinated me enough to write and try it for myself.
I had set up my browser so that it could load the extension from my local machine. I followed the instructions from the documentation and created some compulsory files. Now, I could start coding the actual extension using the bookmarks API.
But wait. What if there is some problem with the setup? What if I missed something critical in the documentation? I needed to be sure before I actually did some work and spent time creating something only to realize that it couldn’t possibly work.
That’s when I felt the need to create a ‘Hello World’ extension.
I think that the Hello World program is not so much of a first program as it is a reassurance that things work. That if I do it properly, my program can work too.
Problem 1: Solved
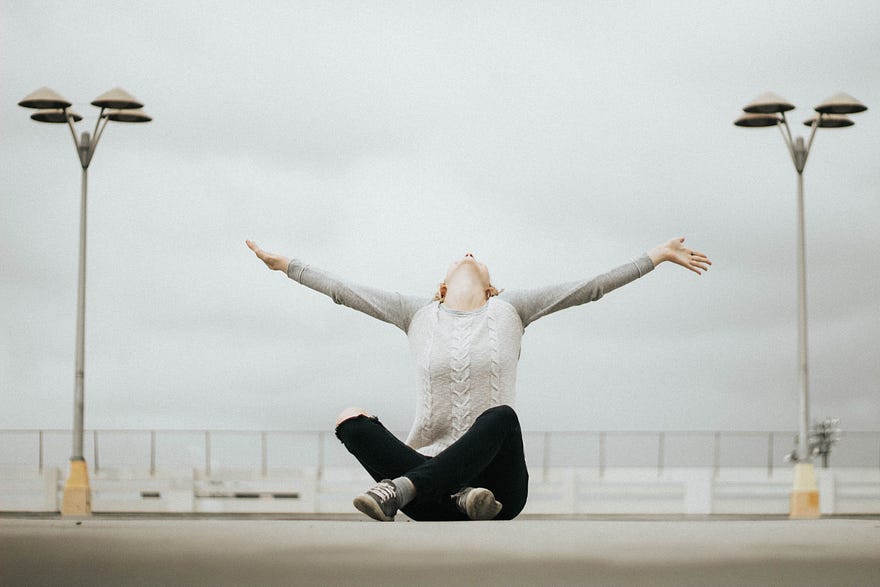
This is what I came up with that night:
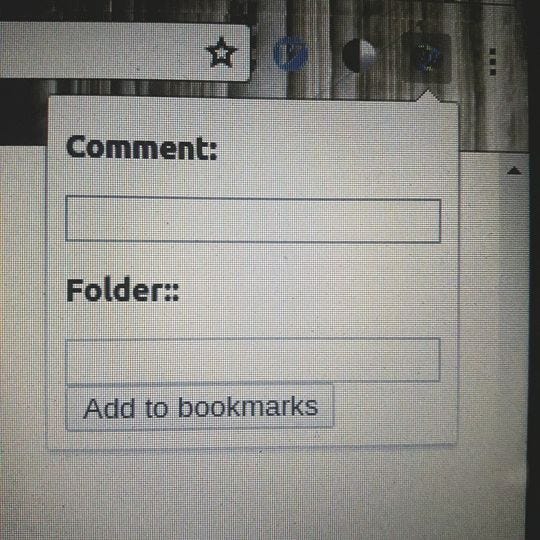
I decided that the webpage title serves as a good default title for the bookmark as well. Therefore, I didn’t add the title field in the popup.
At the time of writing, the code is not well documented or modularized or anything. It just works. I intend to do that in the future. Doing that will be my real programming lesson.
Problem 2: Unsolvable?
As far as I looked, Chrome does not provide any way to generate and download text files to the user’s local machine using bookmarks. This means that we cannot download the bookmarks either 😦.
Override Pages
The documentation itself presented me with a solution.

After reading that, I thought, that’s what I am going to do.
I am going to override the bookmark page with my own custom-designed bookmark manager page.
In it, the bookmarks would not be displayed in selectable tabs as they are now.
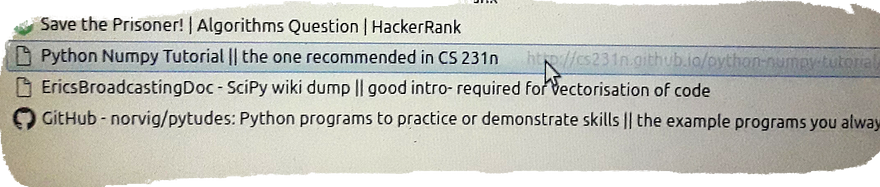
It would be better if the design had simple text for each bookmark, just as most other webpages do, (say, the one you are reading for example). Each bookmark in the text should have the following format:
* Title- <bookmark_title>
* Comment- <bookmark_comment>
* URL- <bookmark_url>
I have not yet created this page but I do intend to do it in the near future.
Thanks for reading!